NetWave CGI protocol from IP cameras
Many of the network IP cams that can be found on the market use the NetWave protocol, based on CGI commands to obtain video images through an internal http server. In this article I will show how to build a simple application to control these cameras, which are typically used for surveillance and can make scan motions in addition to providing video images.
Here you can download the NetWave CGI command reference, and in this other link you can download the IPCameraDemo project source code with the sample application to control the camera, written in C# with Visual Studio 2013.
I have divided the project into three parts, IPCameraDemo is the main program, IPCameraInterfaces contains a common interface used to control different types of IP cameras, and NetWaveCamera is the implementation of this interface for NetWave cameras that use this protocol.
The camera has an internal http server and is controlled by CGI commands. Normally, the access is protected and you must provide a username and password, which are passed as parameters in the requests that you send to the camera. Depending on the type of user you will provide, will be available more or less commands.
Basically, we have three groups of commands:
- Control commands: These are used to obtain information on the camera configuration or to set the value of these parameters, such as the resolution, brightness or contrast, and to move the camera in the case that it is a robotized one.
- Security commands: Allows configuring users and access levels.
- Video commands: To obtain pictures or video streams from the camera.
The urls to access the camera are built according to the following scheme:
<Camera URL>/<CGI command>?<parameter1>=<value>[;<parameter2>=<value>]*
If the camera uses access protection, you must always provide the authentication parameters, user for the user name and pwd for the password, which will determine if you have permission to execute the command.
Camera configuration
To read the camera settings, you have to use the get_camera_params.cgi command. This command returns a text string with the parameters in the format <name>=<value>; separated by a line break. The returned parameters are as follows:
- Resolution: The value 8 indicates a resolution of 320x200 pixels, and 32 is for a resolution of 640x480.
- Brightness: Returns the brightness level of the camera, is an integer between 0 and 255.
- Contrast: The contrast level, an integer between 0 and 6.
- Mode: 0 indicates 50Hz, 1 is for 60Hz, and 2 for outdoor mode.
- Flip: Indicates whether the image is inverted. This is a parameter consisting of two bits, where 0 indicates that the image is not inverted, 1 indicates horizontal rotation, 2 vertical rotation, and 3 is for both horizontal and vertical.
The opposite command, to set the value of these parameters, is camera_control.cgi. You must pass the parameters in the format param=<parameter name>&value=<parameter value>. The parameters are coded as follows: 0 is for the resolution, 1 for brightness, contrast is 2, 3 is for the mode, and 5 to invert the image. For example, the following command will change the brightness setting to its maximum value: camera_control_cgi?param=1&value=255.
Motion control
The robotic cameras that allow movement have a similar aspect as those in the image:
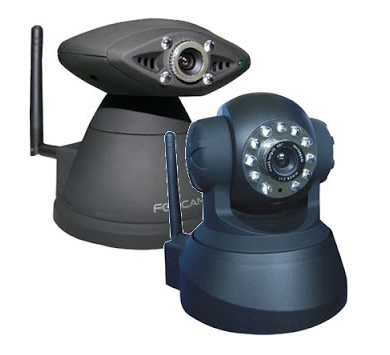
These cameras allow right, left, top and down movements, in addition to being able to perform complete scanning across the viewing area through a single command.
To make a move you have to use decoder_control.cgi command, with the command parameter that indicates the movement direction coded as an integer, in the format decoder_control.cgi?command=<value>. The most common values are as follows:
- 0 and 1: Start or stop the upward movement.
- 2 and 3: Start or stop the downward movement.
- 4 and 5: Start or stop the movement to the left.
- 6 and 7: Start or stop the movement to the right.
- 25: Move the camera to the default position in the center.
- 26 and 27: Start or stop the vertical scanning.
- 28 and 29: Start or stop the horizontal scanning.
Obtaining images from the camera
The command used to obtain a snapshot in jpg format, is snapshot.cgi. You can pass as a parameter the name of the file to download with the optional next_url parameter.
These are the commands that I used in the sample project that accompanies this article. There are many other commands with which you can experience following the reference manual.
IPCameraDemo application
Let's make a small summary of how works the sample program accompanying the article, specifically the library NetWaveCamera which is responsible of controlling the camera. First, we will examine the IIPCamera interface, in the IPCameraInterfaces library, which contains the properties and functions called from the main program:
public interface IIPCamera
{
int Width { get; set; }
int Height { get; set; }
int Fps { get; set; }
bool NeedsAuthentication { get; set; }
void ShowAuthenticationDialog();
void ShowCameraConfiguration();
void Start();
void Close();
event Action<Bitmap> OnNewImage;
void ReleaseEvents();
}
The Width and Height properties allow you to change the image resolution. In this case only the resolutions of 320x200 and 640x480 are supported. With Fps you can control the sampling rate of the camera.
NeedsAuthentication indicate whether you must provide username and password to access the camera, and ShowAuthenticationDialog display a dialog box for entering this data. This dialog box is implemented in the AuthenticationParameters form of the NetWaveCamera project.
ShowcameraConfiguration presents a no modal dialog box with the camera controls: brightness, contrast, flip and movement, implemented in the CameraConfiguration form of the NetWaveCamera project.
To start and stop the capture, you have to use the Start and Close methods, reading the images will be done asynchronously in a separate task, and the images will be passed to the caller program as a Bitmap by the OnNewImage event. You can release the event using the method ReleaseEvents.
To send a control command, build the command url and use the HttpWebRequest class to send it to the camera using the GetResponse method:
string url = _strUrl + (_strUrl.EndsWith("/") ? "" : "/") +
"camera_control.cgi?param=0&value=" +
_nResolution.ToString();
HttpWebRequest wrq = (HttpWebRequest)HttpWebRequest.Create(url);
wrq.Method = "GET";
wrq.Headers.Add("Authorization", "Basic " + Convert.ToBase64String(enc.GetBytes(userpwd)));
WebResponse wr = wrq.GetResponse();
I add an Authorization header to the call to access control, so it is not necessary to pass the username and password in the url parameters.
To read an image, follow the same procedure, obtaining a Stream with the image data:
string url = _strUrl + (_strUrl.EndsWith("/") ? "" : "/") +
"snapshot.cgi?user=" + _strName + "&pwd=" + _strPassword;
HttpWebRequest wrq = (HttpWebRequest)HttpWebRequest.Create(url);
wrq.Method = "GET";
wrq.Headers.Add("Authorization", "Basic " + Convert.ToBase64String(enc.GetBytes(userpwd)));
WebResponse wr = wrq.GetResponse();
Stream receiveStream = wr.GetResponseStream();
if (OnNewImage != null)
{
OnNewImage(new Bitmap(receiveStream));
}
In this case, I have passed the username and password as parameters in the url, in addition to add the authorization header.
As you can see, it is very easy to make a program to control these cameras from any device. Now it is up to you to think ways to use it in your applications.